How to Fix "SyntaxError: Cannot use import statement outside of a module" Error in Node
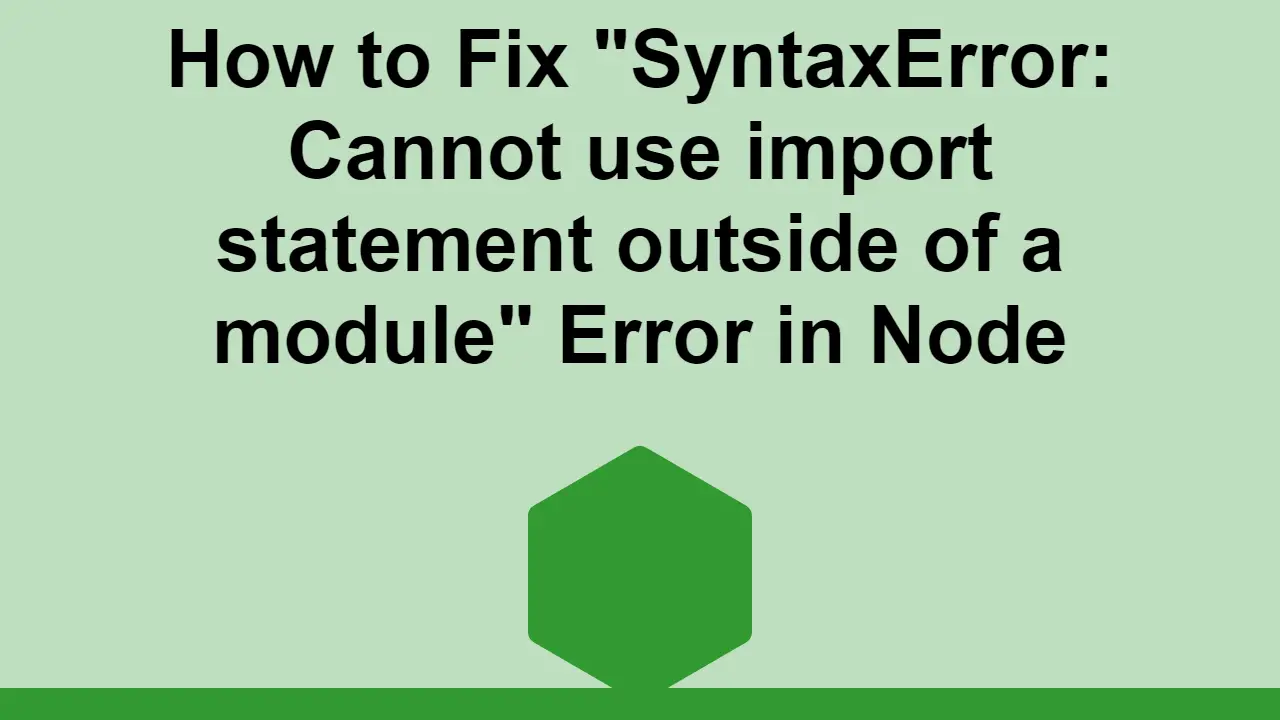
Table of Contents
One of the most confusing parts of the Node ecosystem is that it contains two different module systems, ESM (ECMAScript Modules) and CommonJS. This is a problem because the two module systems are incompatible with each other.
While working in Node, you might come across the following error:
BASHSyntaxError: Cannot use import statement outside a module
In this post, we'll learn more about the "SyntaxError: Cannot use import statement outside a module" error and how to fix it in Node.
How to fix SyntaxError: Cannot use import statement outside a module
The error "SyntaxError: Cannot use import statement outside a module" is caused by the fact that the file you're trying to import is using the ESM module system, while the file you're trying to import it into is using the CommonJS module system.
For example, let's say you have a file called index.js
that uses the CommonJS module system but you try to use the import
keyword:
JAVASCRIPTimport { foo } from './foo.js';
This will throw the error "SyntaxError: Cannot use import statement outside a module" because the index.js
file is using the CommonJS module system.
JavaScript ESM uses the import
and export
keywords to include and make available code from other modules, respectively. CommonJS uses the require()
function to include code from other modules, and the module.exports
object to make code available to other modules.
To fix this, you will either have to continue using the CommonJS module system and use the require
keyword:
JAVASCRIPTconst { foo } = require('./foo.js');
Or you will have to convert your entire project to use the ESM module system and use the import
keyword:
JAVASCRIPTimport { foo } from './foo.js';
How to convert a CommonJS project to use the ESM module system
To convert your Node project to use the ESM module system, you will need to open your package.json
file and add the following property:
JSON{
"type": "module"
}
By setting the type
property to module
, you are telling Node that your project uses the ESM module system.
After you do this, you will be able to use the import
keyword in your project:
JAVASCRIPTimport { foo } from './foo.js';
Conclusion
In this post, we learned about the "SyntaxError: Cannot use import statement outside a module" error and how to fix it in Node.
More specifically, this is caused when you try and mix the ESM and CommonJS module systems in the same project. To fix this, you will either have to continue using the CommonJS module system and use the require
keyword or switch over entirely to the ESM module system and use the import
keyword.
Thanks for reading!
- How to Install Node on Windows, macOS and Linux
- Managing PHP Dependencies with Composer
- Getting Started with Express
- Git Tutorial: Learn how to use Version Control
- How to deploy a .NET app using Docker
- How to deploy a PHP app using Docker
- How to deploy a Deno app using Docker
- How to deploy an Express app using Docker
- Using Puppeteer and Jest for End-to-End Testing
- How to Scrape the Web using Node.js and Puppeteer
- Build a Real-Time Chat App with Node, Express, and Socket.io
- Getting Started with Vuex: Managing State in Vue