How to Fix TypeError: list indices must be integers or slices, not tuple in Python
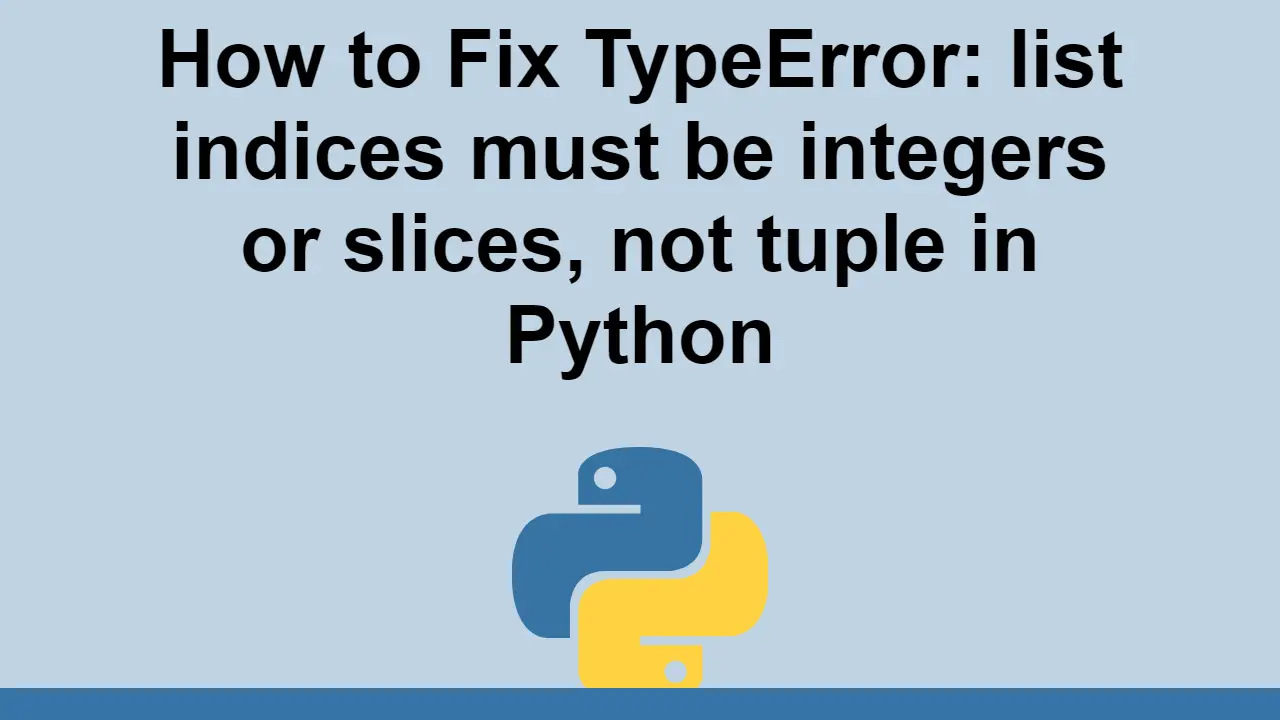
When you're working in Python, especially with lists and tuples, you might come across this error:
BASHTypeError: list indices must be integers or slices, not tuple
This error is caused by the fact that you're trying to access a list element by a tuple.
In this post, we'll look and some example code that causes this error and how to fix it.
How to fix TypeError: list indices must be integers or slices, not tuple
Before we learn how to fix it, let's look at some example code that causes this error:
PYTHONlocations = [
["New York", "USA"]
["Paris", "France"]
]
london = ["London", "UK"]
locations.append(london)
print(locations)
Depending on your compiler, you might even already get the solution to this problem:
BASH<string>:2: SyntaxWarning: list indices must be integers or slices, not tuple; perhaps you missed a comma?
Traceback (most recent call last):
File "<string>", line 2, in <module>
TypeError: list indices must be integers or slices, not tuple
It says it on the first line of this error, that perhaps you missed a comma, and indeed we did.
By defining locations
without a comma, we're creating a list of lists, instead of a list of tuples.
That distinction is the root of the problem.
All we need to do is add a comma so that the compiler understands that this is a list of tuples:
PYTHONlocations = [
["New York", "USA"],
["Paris", "France"]
]
london = ["London", "UK"]
locations.append(london)
print(locations)
As expected, this now works without errors.
BASH[['New York', 'USA'], ['Paris', 'France'], ['London', 'UK']]
Conclusion
In this post, we learned how to fix the problem of TypeError: list indices must be integers or slices, not tuple.
Simply ensure that you are using a list of tuples, instead of a list of lists, which can be done by adding a comma to separate the different tuples.
Thanks for reading!
- Getting Started with TypeScript
- Getting Started with Solid
- Getting Started with Svelte
- Getting Started with Express
- How to Serve Static Files with Nginx and Docker
- How to deploy a .NET app using Docker
- How to deploy a PHP app using Docker
- How to deploy a MySQL Server using Docker
- Using Puppeteer and Jest for End-to-End Testing
- Getting Started with Handlebars.js
- Learn how to build a Slack Bot using Node.js
- Getting Started with React